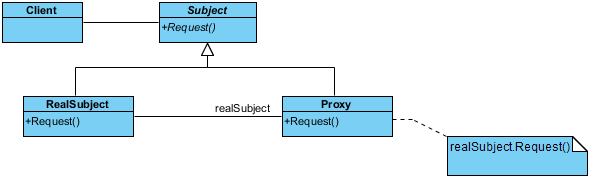
This is a UML class diagram example for the Proxy design pattern.
Purpose
Allows for object level access control by acting as a pass through entity or a placeholder object.
Use When
- The object being represented is external to the system.
- Objects need to be created on demand.
- Access control for the original object is required.
- Added functionality is required when an object is accessed.
Import into your Project
Open diagram in Visual Paradigm [?]Copy the URL below, paste it in the Open Project windows of Visual Paradigm and press Enter to open it |
Posted by: